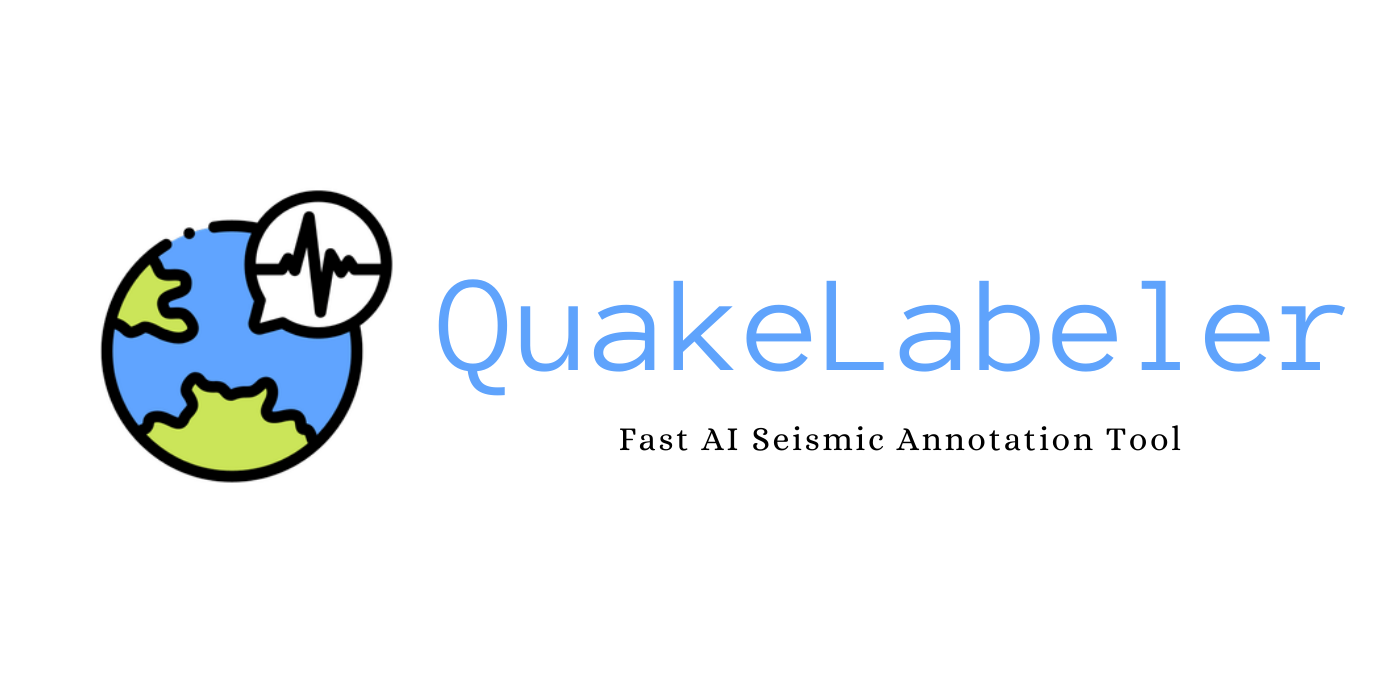
Classes¶
quakelabeler
defines the following base classes:
QuakeLabeler¶
- class quakelabeler.classes.QuakeLabeler(query, custom)¶
Quake Labeler
class enables to automatically label ground truth. AQuakeLabeler
object contains Class attributes that design and create seismic datasets by custom settings. QuakeLabeler runs a series of methods to automatic generate datasets:Define research region and time range
Define automation dataset options
Retrieve seismograms from data center
Pre-process (optional): detrend, denoise, resampling, filter
Transfer waveforms to standard labels (Annotation)
Post-process (optional): crop, add noise, generate input/output channels
Save label recordings as independent CSV files
Export distribution histogram
This is intended to be the core QuakeLabeler functionality, without any reference to the command line interface. The original intent was to allow this to run independently, eg. from a script or interactive shell.
- Parameters
params (dict) –
params containing research region and time range options in a dictionary which receive from interactive shell or scripts. params options include:
station region
event time range
event magnitude limits(optional)
arrival limits(optional)
recordings (dict) –
Event-based records (i.e., arrival information) request from ISC bulletin. Note that these recordings do not gurantee they are downloadable from certain data centers. recordings includes:
event ID
event magnitude
phase name
arrival time
original event time
event location (optional)
- search_catalog(clientname='IRIS')¶
Availble data source check for data centers. If the desired data center does not have available seismograms, this module will reminder user to change data center.
- search_stations(clientname='IRIS')¶
Search all available station in the target research region. This method accepts rectangular or circular range options.
- Parameters
clientname (str, optional) – Data center name. The default is “IRIS”.
- Returns
inventory – Return an Inventory object which stored available stations.
- Return type
Inventory object
- judge_time_range(thread, t1, t2, clientname='IRIS')¶
Check specific seismogram’s time window. Method to examine if there’s available waveform from certain data center for download in the target time range.
- waveform_timewindow(thread, sample_points=3000)¶
Calculate sample’s startime and endtime. Method to ensure retrieve enough time length waveform. :param thread: thread stores a specific seismogram information. :type thread: dict :param sample_points: Waveform length. The default is 50*60 = 3000. :type sample_points: int, optional
- Returns
starttime (UTCTime) – Start time for this waveform.
endtime (UTCTime) – End time for this waveform.
- fetch_waveform(thread, clientname='IRIS')¶
Retrieve a target stream of waveforms from specific data center. This stream can includes multiple-component seismic traces which from only one station with one event. They can be spilt as single trace mode or keep as a 3-C sample or multiple-component sample. Note that for now we remove those low-sampling-rate (<1.0Hz) samples which might not help our project. :param thread: Waveform information recording. :type thread: dict :param clientname: Name of data center. The default is “IRIS”. :type clientname: str, optional
- Returns
st (Obspy Stream Object) – Downloaded waveform which save as a obspy.stream object.
`No data available for request.` (str) – Failed request message.
- creatsamplename(stream)¶
Creat filename for each sample Creat filenames for each available waveform. :param stream: Availble data stream waited to be transferred to label. :type stream: Obspy stream object
- Returns
filename – creat a name for the waveform.
- Return type
str
- output_bell_dist(npts, it, window)¶
Create bell-like output channel Use bell-like (Gaussian) distribution to form output labels.
- output_rect_dist(npts, it, window)¶
Create rectangular output channel Use rectangulardistribution to form output labels. The positive data points = 1.0 The negative data points = 0.0
- single_sample_export(st, filename, pick_win=100, detect_win=200)¶
Export sample in single channel mode
- multi_sample_export(st, filename, pick_win=100, detect_win=200)¶
Export sample in multiple channel mode
- fetch_all_waveforms(records, clientname='IRIS')¶
Auto fetch seismograms to produce samples This module manage all potential waveforms as threads. Retrive waveform from specific data centers, revise trace by customized parameters and produce required samples continuous until reach the volume.
- This function contains:
Load/Import: Requests to data centers to find available data;
Custom: Use user’s preferrence parameters to form the samples;
Process: (resample, filter, denoise, add noise);
Revise: fix trace format to satify datasets;
Label: Annotate arrival time and phase name;
Export: Save samples in certain file format.
- Parameters
records (list) – records saves all potential downloadable waveform.
clientname (str, optional) – The default is “IRIS”. Specific data center’s name.
- Returns
available_samples – Return every samples in the datasets.
- Return type
list
- subfolder(trainratio=0.8)¶
Split dataset Divide dataset as a training dataset(80%) and a validation dataset(20%)
- csv_writer()¶
Method to export information of the dataset.
- stats_figure()¶
Output statistical figures. Method to plot magnitude distribution.
- waveform_display()¶
Plot generated seismic label. Method to display generated seismic label case to show how the label looks like.
Interactive¶
- class quakelabeler.classes.Interactive¶
Interactive tool for target stations and time range Receive user’s interest search options from command line inteface (CLI). Automatic search arrivals associated to events in the ISC Bulletin on the input stations and time range.
- Options includes:
station region
time range
magnitude range
phase names
ISC Bulletin: arrivals search International Seismological Centre (20XX), On-line Event Bibliography, https://doi.org/10.31905/EJ3B5LV6
- Parameters
params (dict) – params saves all customized options which defines the research region and time. params contains region latitude and longitude, start time and end time, magnitudes(optional), etc.
- input_stn_stn()¶
Station Region Mode: <STN>:
- input_stn_rect()¶
Input station region in rectangular mode
- input_stn_circ()¶
Input station region in circular mode
- input_time()¶
Input <event-time-range> params
- input_mag()¶
Input <event-magnitude-limits> (Optional) params
- select_stnsearch()¶
Choose mode for station region search
- input_event_rect()¶
Event Region Mode: <RECT>: Rectangular search of stations
- input_event_circ()¶
Event Region Mode: <CIRC>: Rectangular search of Events
- beginner_mode(field=1)¶
Run beginner mode User can use this method to select one example region to create dataset
- advanced_mode()¶
Run advanced mode This method is to run a command line interactive module to let user to design their own research region and time range settings.
- select_mode()¶
Mode selection :returns: * beginner_mode (function) – Run beginner mode self.beginner_mode() with default parameters.
advanced_mode (function) – Run advanced mode self.advanced_mode() with various customized options.
CustomSamples¶
- class quakelabeler.classes.CustomSamples(default_option=False)¶
Command line interactive tool for custom dataset options Input paratemter to standardize retrived waveform. :param default_option: if default_option is True, apply default options. :type default_option: bool
- define_dataset()¶
Dataset options
- Returns
custom_dataset – custom_dataset returns a dict which saves all dataset options.
- Return type
dict
- define_waveform()¶
Waveform options Receive waveform format
- Returns
custom_waveform – Returns a dictionary which saves all waveform options.
- Return type
dict
- define_export()¶
- Export options for dataset
- Receive interactive arguements to choose export format, include:
export_type: SAC, Mini-Seed, NPZ, MAT, etc.
export_inout: True/False separate input / output traces
export_out_form: gaussian / peak / rect
#. export_arrival_csv: True / False save arrival information as a independent csv file #. export_stats: True / False
- Returns
custom_export – Export options for dataset.
- Return type
dict
QueryArrival¶
- class quakelabeler.classes.QueryArrival(**kwargs)¶
Auto request online arrivals catalog This class fetch users’s target arrivals from ISC Bulletin website.
References
[1] International Seismological Centre (20XX), On-line Bulletin, https://doi.org/10.31905/D808B830
- find_all_vars(text, *args)¶
Store all arrival information This method save all fetched information into recordings:
EVENTID
STA
PHASE NAME
ARRIVAL DATE
ARRIVAL TIME
ORIGIN DATE
ORIGIN TIME
EVENT TYPE
EVENT MAG